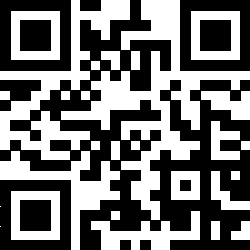
QR codes is one of the most popular formats of 2D codes. It can be useful in various projects. The great advantage is any modern smartphone can scan it. QR codes can be used as a bridge between physical objects and digital system.
Native support of QR Codes in Laravel
Currently, Laravel doesn’t offer the ability to generate QR codes. We can utilize Composer to install the package we need for this purpose. In this article, I’ve used this wrapper: Simple-QrCode.
Simple QrCode functionality
QR codes are encoded text transformed into a graphical form. If we want to generate a QR code in our application, we can use the generate function and pass the information to be transformed as an argument:
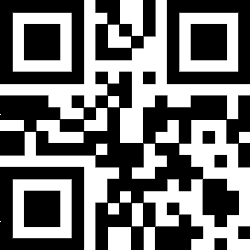
//Generate QR Code with encoded message "Hello World!" QrCode::generate('Hello World!');
The code can be saved as a graphic file or used in Blade templates on the website. Currently, supported graphic formats for QR codes on this wrapper are: png, eps, and svg.
Not only the content of the QR code can be changed, but also its appearance. This wrapper supports method chaining and offers many functionalities that allow users to change, among others: the color of the code, the background color, the shape of the code, the size of the image, and even allows adding a graphic logo as part of the QR code.
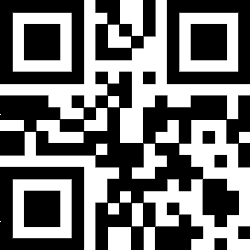
//Generate QR Code of size 250px x 250px QrCode::size(250)->generate('Hello World!');
//Generate QR Code of green color QrCode::color(0, 255, 0)->generate('Hello World!');
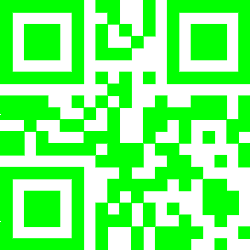
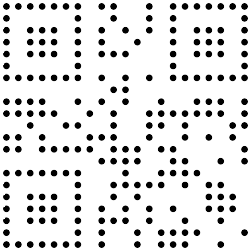
//Generate QR Code with dots instead of squares QrCode::style('dot')->generate('Hello World!');
//Generate QR Code including error correction QrCode::errorCorrection('H')->generate('Hello World!');
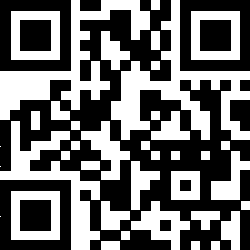
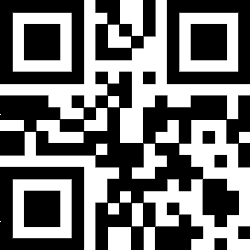
//Generate QR Code in PNG format QrCode::format('png')->generate('Hello World!');
//Or we can mix it all up QrCode::size(250) ->color(0, 255, 0) ->style('dot') ->errorCorrection('H') ->format('png') ->generate('Hello World!');
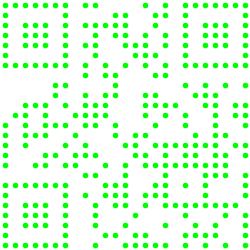