TypeScript is a superset of JavaScript, which adds optional static typing and other features on top of JavaScript. It enhances productivity and improves code quality, but like any technology, apart from its strengths, it has some limitations.
It requires declaring the data type of variables when defining them, but helps to predict how the code will run.
interface Person { name: { firstName: string; lastName: string; }; age: number; } const getPersonName = (person: Person | null) => { if (person === null) { return; } // if person is not null, it should contain a name property // containing firstName and lastName, which are strings return `${person.name.firstName} ${person.name.lastName}`; }
Typescript has more error messages during development, but it allows to catch potential issues before deployment to production, so getting runtime errors is less likely.
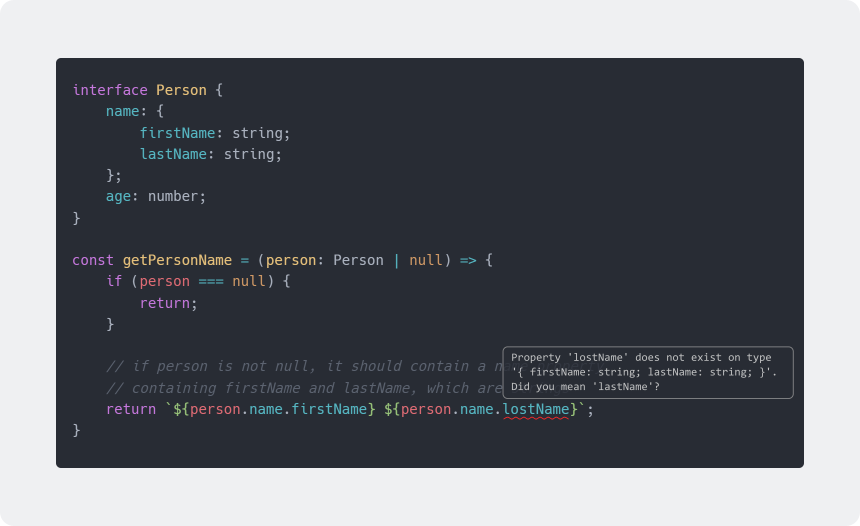
TypeScript usually requires more code due to type annotations and stricter rules, but offers better autocomplete support because of the type definitions.
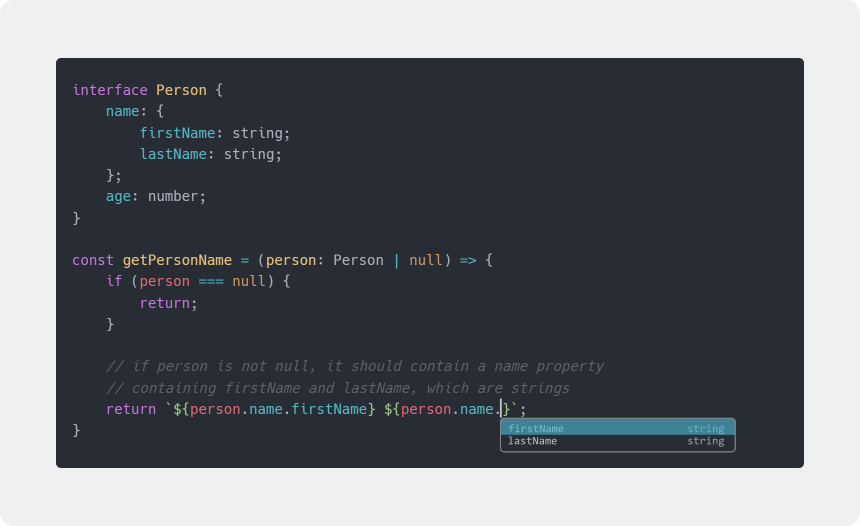
Conclusion
Code written in TypeScript is usually longer, but easier to maintain and of higher quality. It’s clear that TypeScript’s benefits outweigh its drawbacks.